Missing triangle in BVH
Recently I was applying Bounding Volume Hierachy to a Whitted-style Raytracing system. The idea of BVH and implementation are well described in Ray Tracing in the Next Week by Peter Shirly. It’s a really great book. And here’s the link https://raytracing.github.io/books/RayTracingTheNextWeek.html
One key component in BVH is AABB (Axis-Aligned-Bounding-Box) intersects with ray. People usually adopt Slab method to address this problem. To be more specific, computing the ray-AABB intersection along 3 different axis, if these intervals produces an overlapping region, then the ray intersects with AABB and vice versa.
1 | compute (tx0, tx1) |
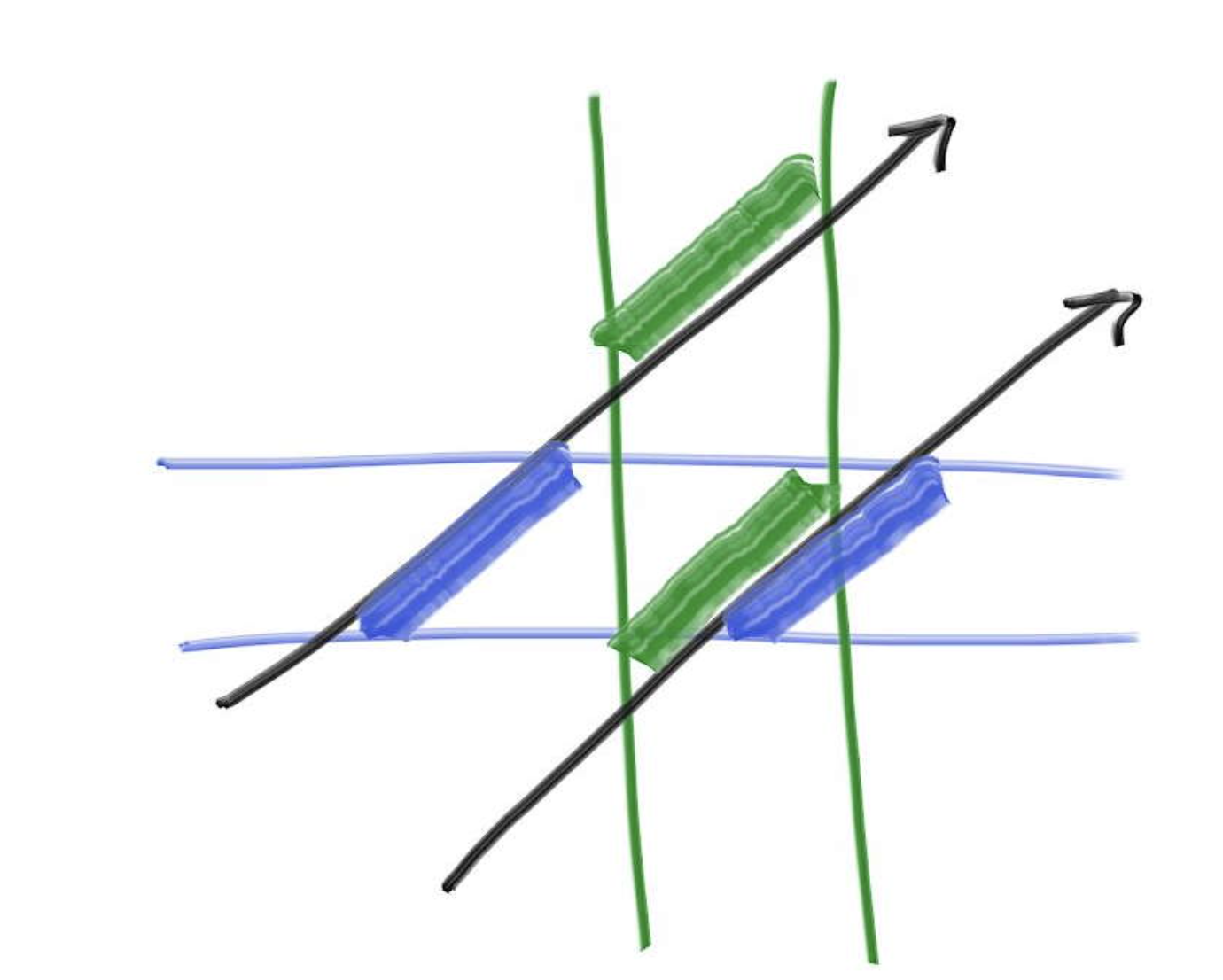
After applying this method to the scene rendering, I lost some of the triangles in the scene. However, all spheres are kept intact. For example, here’s what I got after applying BVH and the latter is before BVH.
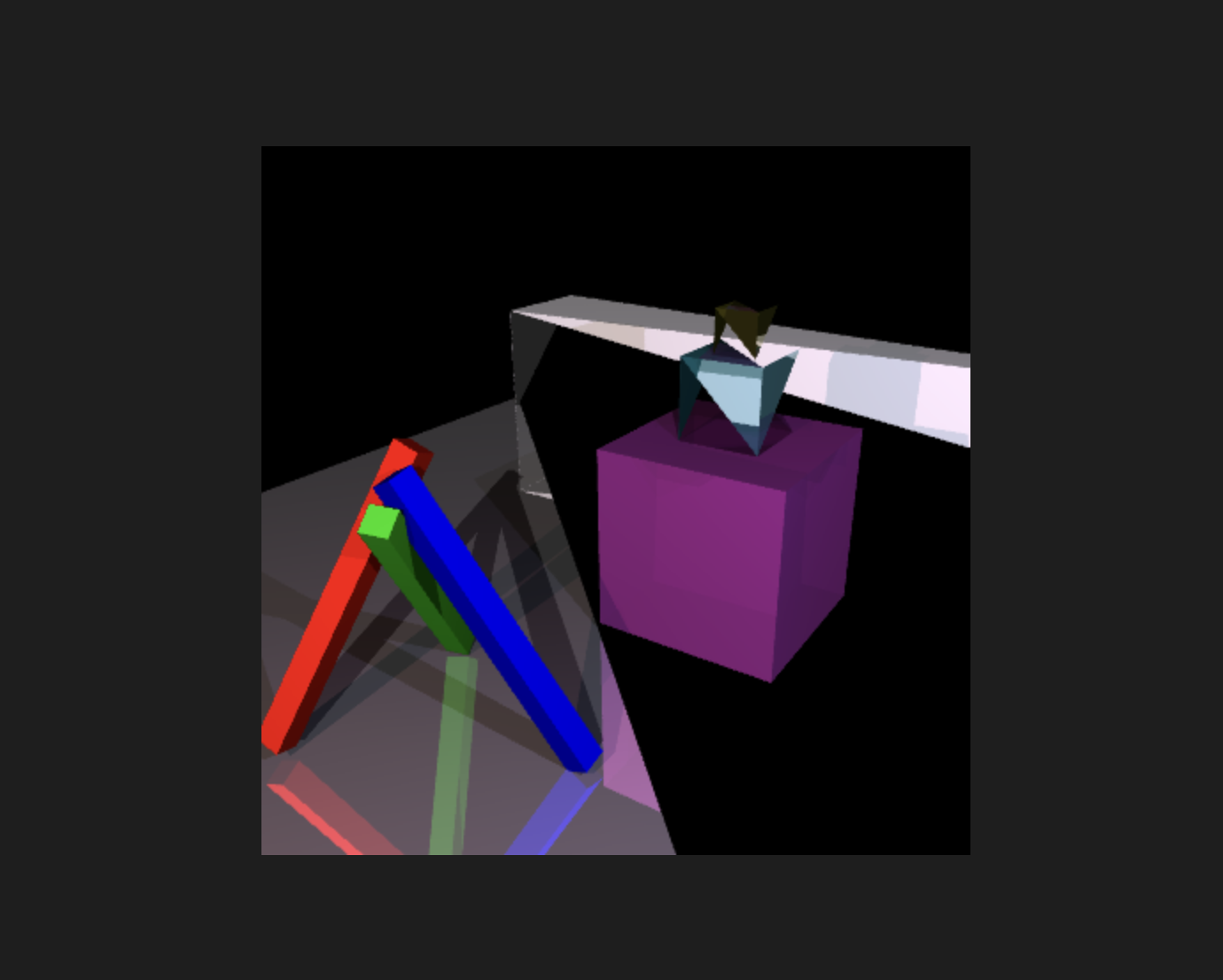
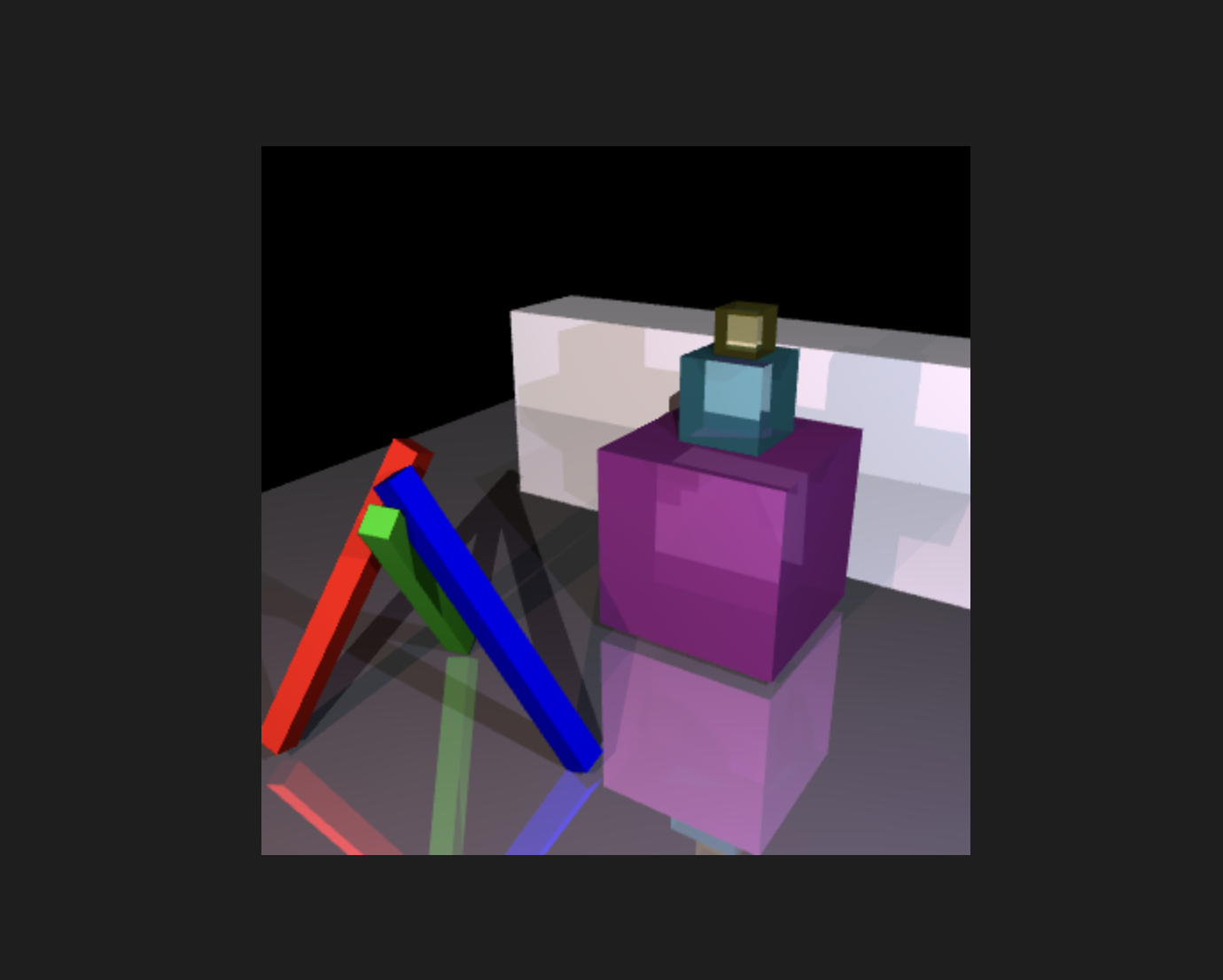
After couple hours of step_into
debugging, I found the reason to be as follows:
For spheres, the Bouding box will always have some length along 3 axis. However, this isn’t necessarily the case for AABB of triangles. ==Bounding Box of triangles can fall back to plane if the triangle is parallel to either xy, yz or xz plane== .
Let’s say a triangle is parallel to the
xz
plane, so the 3 vertices has the samey
value. And this makesty0
andty1
to be the same in above computation, and thus resulting no overlapping region for other 2 axis. i.e. The bounding box doesn’t intersect with the triangle (which is a false statement)
To address this, simply add some thickness to each axis while building bounding box for triangles. In my case, I use 1e-3
, and boom, problem solved!
1 | //in triangle class |